1 项目简介
1.1 技术架构
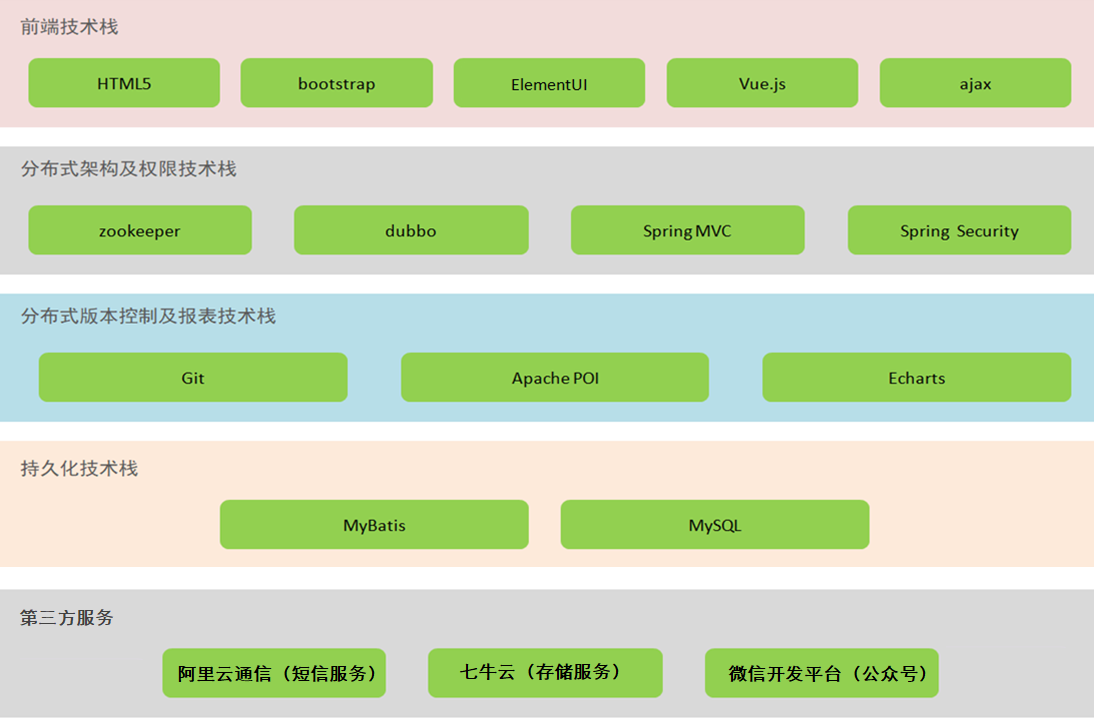
1.2 功能架构
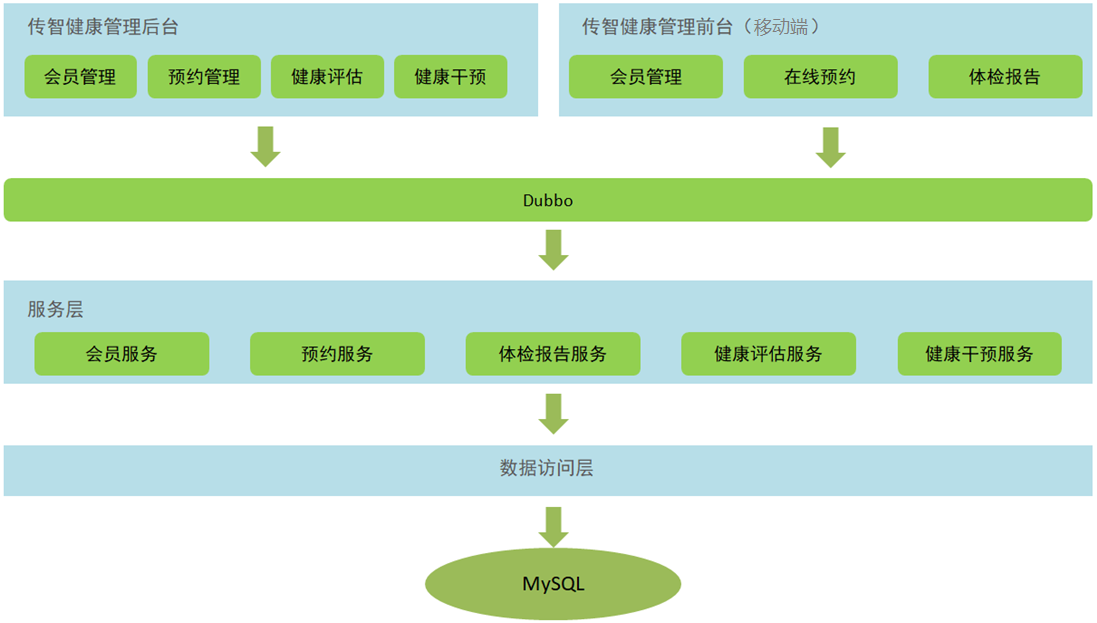
1.3 项目结构
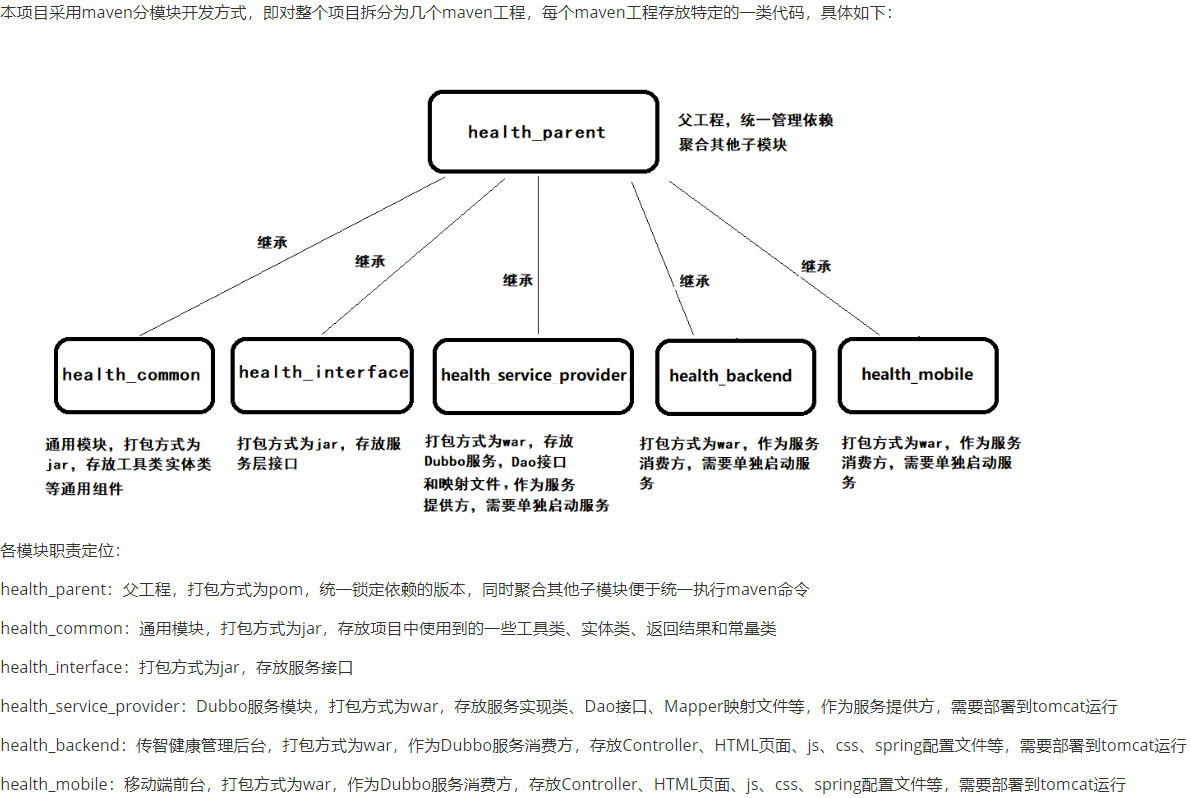
1.4 项目启动
模块
health_backend controller webapp tomcat7:82 启动2
health_common constant、entity、pojo、utils
health_interface service
health_jobs tomcat7:83
health_mobile controller webapp tomcat7:80
health_service_provider service.impl、mapper tomcat7:81 启动1
模块
health_backend redis、zookeeper http://localhost:82/pages/main.html
health_common
health_interface
health_jobs redis、jobs
health_mobile redis、zookeeper http://localhost/pages/index.html
health_service_provider mysql、redis、zookeeper
2 图片上传:七牛云kodo
2.1 介绍
Java SDK 属于七牛服务端SDK之一,主要有如下功能:
提供生成客户端上传所需的上传凭证的功能
提供文件从服务端直接上传七牛的功能
提供对七牛空间中文件进行管理的功能
提供对七牛空间中文件进行处理的功能
提供七牛融合CDN相关的刷新,预取,日志功能
提供七牛视频监控QVS设备管理、流管理等功能
2.2 依赖
<dependency>
<groupId>com.qiniu</groupId>
<artifactId>qiniu-java-sdk</artifactId>
<version>7.2.0</version>
</dependency>
2.3 工具类
/**
* 七牛云工具类
*/
public class QiniuUtils {
public static String accexsKey = "";
public static String secrxtKey = "";
public static String bucket = "qiniutest";
public static void upload2Qiniu(String filePath,String fileName){
//构造一个带指定Zone对象的配置类
Configuration cfg = new Configuration(Zone.zone0());
UploadManager uploadManager = new UploadManager(cfg);
Auth auth = Auth.create(accessKey, secretKey);
String upToken = auth.uploadToken(bucket);
try {
Response response = uploadManager.put(filePath, fileName, upToken);
//解析上传成功的结果
DefaultPutRet putRet =
new Gson().fromJson(response.bodyString(), DefaultPutRet.class);
} catch (QiniuException ex) {
Response r = ex.response;
try {
System.err.println(r.bodyString());
} catch (QiniuException ex2) {
//ignore
}
}
}
//上传文件
public static void upload2Qiniu(byte[] bytes, String fileName){
//构造一个带指定Zone对象的配置类
Configuration cfg = new Configuration(Zone.zone0());
//...其他参数参考类注释
UploadManager uploadManager = new UploadManager(cfg);
//默认不指定key的情况下,以文件内容的hash值作为文件名
String key = fileName;
Auth auth = Auth.create(accessKey, secretKey);
String upToken = auth.uploadToken(bucket);
try {
Response response = uploadManager.put(bytes, key, upToken);
//解析上传成功的结果
DefaultPutRet putRet =
new Gson().fromJson(response.bodyString(), DefaultPutRet.class);
System.out.println(putRet.key);
System.out.println(putRet.hash);
} catch (QiniuException ex) {
Response r = ex.response;
System.err.println(r.toString());
try {
System.err.println(r.bodyString());
} catch (QiniuException ex2) {
//ignore
}
}
}
//删除文件
public static void deleteFileFromQiniu(String fileName){
//构造一个带指定Zone对象的配置类
Configuration cfg = new Configuration(Zone.zone0());
String key = fileName;
Auth auth = Auth.create(accessKey, secretKey);
BucketManager bucketManager = new BucketManager(auth, cfg);
try {
bucketManager.delete(bucket, key);
} catch (QiniuException ex) {
//如果遇到异常,说明删除失败
System.err.println(ex.code());
System.err.println(ex.response.toString());
}
}
}
2.4 测试
/**
* 体检套餐管理
*/
@RestController
@RequestMapping("/setmeal")
public class SetmealController {
@Autowired
private JedisPool jedisPool;
//文件上传
@RequestMapping("/upload")
public Result upload(@RequestParam("imgFile") MultipartFile imgFile){
System.out.println(imgFile);
String originalFilename = imgFile.getOriginalFilename();//原始文件名 3bd90d2c-4e82-42a1-a401-882c88b06a1a2.jpg
int index = originalFilename.lastIndexOf(".");
String extention = originalFilename.substring(index - 1);//.jpg
String fileName = UUID.randomUUID().toString() + extention;// FuM1Sa5TtL_ekLsdkYWcf5pyjKGu.jpg
try {
//将文件上传到七牛云服务器
QiniuUtils.upload2Qiniu(imgFile.getBytes(),fileName);
jedisPool.getResource().sadd(RedisConstant.SETMEAL_PIC_RESOURCES,fileName);
} catch (IOException e) {
e.printStackTrace();
return new Result(false, MessageConstant.PIC_UPLOAD_FAIL);
}
return new Result(true, MessageConstant.PIC_UPLOAD_SUCCESS,fileName);
}
}
-------------------------------------------------------------------------------------------------------------
/**
* 自定义Job,实现定时清理垃圾图片
*/
public class ClearImgJob {
@Autowired
private JedisPool jedisPool;
public void clearImg(){
//根据Redis中保存的两个set集合进行差值计算,获得垃圾图片名称集合
Set<String> set = jedisPool.getResource().sdiff(RedisConstant.SETMEAL_PIC_RESOURCES, RedisConstant.SETMEAL_PIC_DB_RESOURCES);
if(set != null){
for (String picName : set) {
//删除七牛云服务器上的图片
QiniuUtils.deleteFileFromQiniu(picName);
//从Redis集合中删除图片名称
jedisPool.getResource().srem(RedisConstant.SETMEAL_PIC_RESOURCES,picName);
System.out.println("自定义任务执行,清理垃圾图片:" + picName);
}
}
}
}